WordPress Script to Display User Names and Their Post Lists
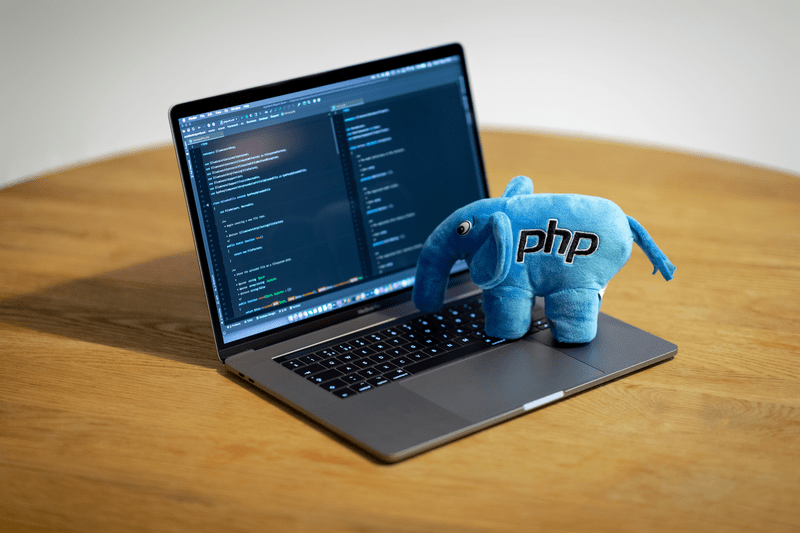
Listing Users and Their Posts on Your Website
On our website, we provide a comprehensive list of contributors, showcasing their individual profiles along with the posts they have authored. Each contributor’s profile includes their nickname, a Gravatar icon for easy identification, and a neatly organized list of all their published posts.
To achieve this, we use a custom shortcode which dynamically generates the list of users and their posts. The contributors’ nicknames are displayed prominently as headings, accompanied by their Gravatar icons. Beneath each nickname, you will find a numbered list of all the posts written by that user, with each post title linked directly to the full article.
This feature not only highlights the valuable contributions of each author but also allows visitors to explore the content created by their favorite writers easily. The design ensures a user-friendly experience, with hover effects that underline nicknames and post titles, enhancing the visual appeal and navigation on the site.
By implementing this functionality, we aim to foster a sense of community and recognition for our contributors while providing a seamless browsing experience for our audience.
This script requires some knowledge of PHP and CSS.
I use fluent snippet plugin for all my functions, js, and CSS:
Step 1. PHP Coding:
function list_all_users_nicknames_and_posts() {
// Get all users
$users = get_users();
// Start output buffering
ob_start();
// Loop through each user and list their nickname and posts
foreach ($users as $user) {
echo '<div class="user-nickname">';
// Get the Gravatar
$gravatar_url = get_avatar_url($user->ID);
// Display Gravatar and nickname with title attribute
echo '<h2><img src="' . esc_url($gravatar_url) . '" alt="' . esc_attr($user->nickname) . '" class="user-gravatar" title="View posts by ' . esc_attr($user->nickname) . '">' . esc_html($user->nickname) . '</h2>';
// Get posts by the user
$args = array(
'author' => $user->ID,
'post_status' => 'publish',
'posts_per_page' => -1 // Get all posts
);
$user_posts = get_posts($args);
if (!empty($user_posts)) {
echo '<ol class="user-posts-list">';
foreach ($user_posts as $post) {
echo '<li><a href="' . get_permalink($post->ID) . '" title="' . esc_attr($post->post_title) . '">' . esc_html($post->post_title) . '</a></li>';
}
echo '</ol>';
} else {
echo '<ol class="user-posts-list"><li>No posts found.</li></ol>';
}
echo '</div>';
}
// Return the buffered content
return ob_get_clean();
}
// Register the shortcode [user_nick]
add_shortcode('user_nick', 'list_all_users_nicknames_and_posts');
Step 2. CSS Coding:
/* Style for the user nickname heading */
.user-nickname {
margin: 20px 0;
padding: 10px;
background-color: #f9f9f9;
border: 1px solid #ddd;
border-radius: 4px;
}
.user-nickname h2 {
margin: 0 0 10px 0;
padding: 0;
font-size: 1.5em;
display: flex;
align-items: center;
}
.user-nickname h2:hover {
text-decoration: underline;
}
.user-gravatar {
border-radius: 50%;
margin-right: 10px;
}
/* Style for the user posts list */
.user-posts-list {
list-style-type: decimal;
margin: 10px 0 0 20px;
padding: 0;
}
.user-posts-list li {
margin: 5px 0;
}
.user-posts-list li a {
text-decoration: none;
}
.user-posts-list li a:hover {
text-decoration: underline;
}
Step 3. Shortcode:
[user_nick]
This will generate a list where each user’s nickname is displayed as a <h2>
heading with their Gravatar icon inline, and their corresponding posts listed below in a numerical list with an underline on hover effect.
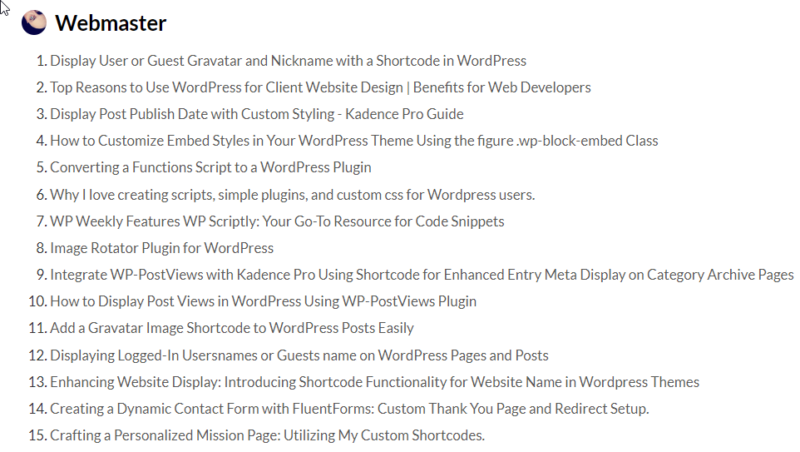
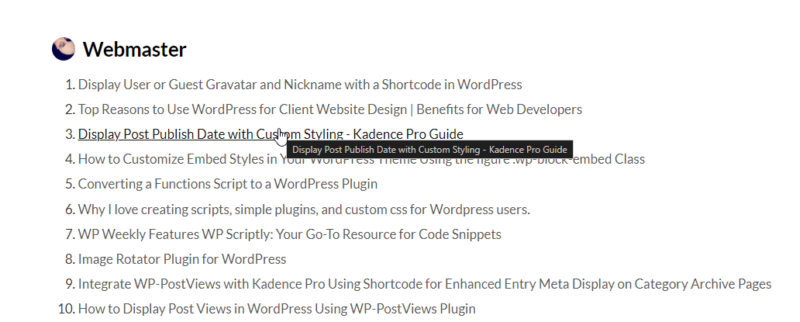
Summary
- Add the PHP code to your
functions.php
or a custom plugin to create the shortcode. - Add the updated CSS to your
style.css
to style the list. - Use the shortcode in your posts or pages to display the list of users and their post.
- Modify the CSS to match your website.
- The Title Attribute has been added for better SEO.
By following these steps, you will have a nicely styled list of all users’ nicknames with their Gravatar icons as <h2>
headings along with their posts in a numerical list, with an underline on hover effect for both the nicknames and the post titles on your WordPress site using the provided shortcode.
Notes:
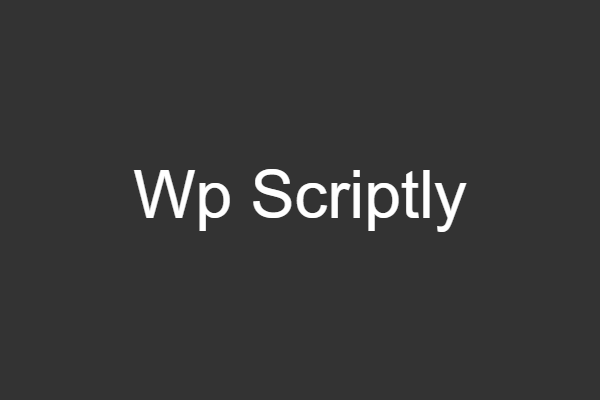
This basic script, CSS style, and plugin are designed to function optimally assuming minimal interference from your theme or other plugins. If conflicts occur or further customization is needed, additional adjustments may be necessary. Please note that this script, CSS style, or plugin must be used AS IS.
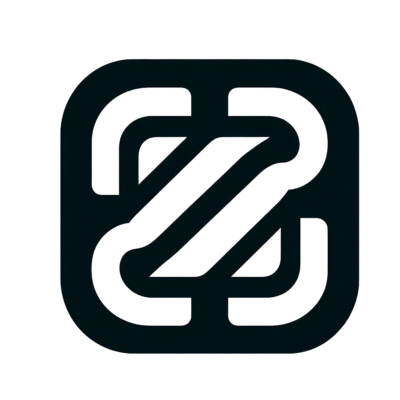
Wp Scriptly
Comments: