Dynamically Insert Ads in WordPress Archive Templates Using Custom Scripts
As someone constantly experimenting with WordPress customization, I wanted to control how and where ads appeared on my archive pages. Instead of relying on ad plugins with bloated features, I took a more efficient route—writing a custom script to insert ads after every 3rd, 5th, and 7th post dynamically. Here’s how I did it and why this solution works like a charm.
The Idea Behind It
My goal was to keep things lightweight and flexible. I wanted the option to insert ad blocks automatically without manually placing them in each post. This meant using a combination of a custom PHP function and a simple shortcode.
I use fluent snippet plugin for all my functions, js, and CSS:
The Custom Script
I created a function that counts posts in the loop and conditionally injects ads at specific positions. This way, when a visitor scrolls through the archive, they see non-intrusive, well-placed ads, seamlessly blending into the layout.
Here’s a simplified version of the code I used:
function kadence_insert_ad_after_posts($post_id) {
global $post_count;
// Initialize counter if not set
if (!isset($post_count)) {
$post_count = 0;
}
$post_count++; // Increment counter with each post
// Insert the ad after the 3rd and 5th posts
if ($post_count == 3 || $post_count == 5 || $post_count == 7) {
echo '<div class="custom-ad">
<h4>Sponsored Ad</h4>
<p>'. do_shortcode('[ADD YOUR SHORTCODE HERE]') .'</p>
</div>';
}
}
add_action('kadence_loop_entry', 'kadence_insert_ad_after_posts', 20);
If you have more than 10 post in your archive page ( this is per page) you can adjust this line to display more:
if ($post_count == 3 || $post_count == 5 || $post_count == 7) {
to
if ($post_count == 3 || $post_count == 5 || $post_count == 7 || $post_count == 10) {
So just add a higher number at the end. || $post_count == 12
New Updated coding
This will place the ads ever 3rd on automatically.
function kadence_insert_ad_after_posts($post_id) {
global $post_count;
// Initialize counter if not set
if (!isset($post_count)) {
$post_count = 0;
}
$post_count++; // Increment counter with each post
// Insert the ad every 3rd post
if ($post_count % 3 == 0) {
echo '<div class="custom-ad">
<h4>Sponsored Ad</h4>
<p>' . do_shortcode('[ADD YOUR SHORTCODE HERE]') . '</p>
</div>';
}
}
add_action('kadence_loop_entry', 'kadence_insert_ad_after_posts', 20);
Please style the ad block to match your website!
*This is my CSS for the ad block.
.custom-ad {
margin: 20px 0;
padding: 15px;
background: #F1F3F4;
text-align: center;
border-top: 2px solid #595957!important;
border-left: 1px solid #DCDCDE!important;
border-right: 1px solid #DCDCDE!important;
border-bottom: 1px solid #DCDCDE!important;
box-shadow: 0px 0px 1px rgb(0 0 0 / 10%), 0px 4px 8px rgb(0 0 0 / 10%)!important;
}
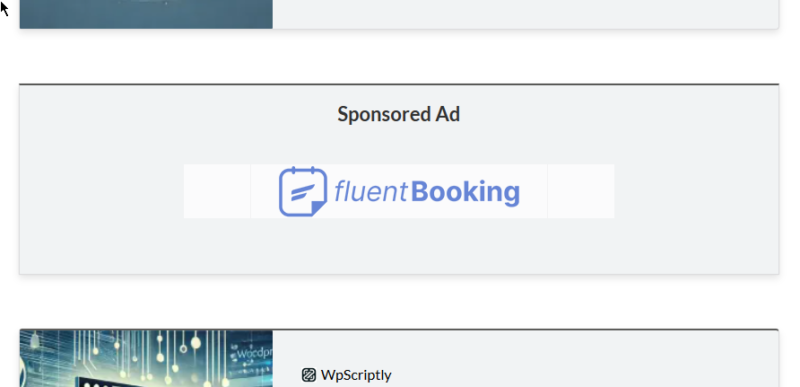
Comments: